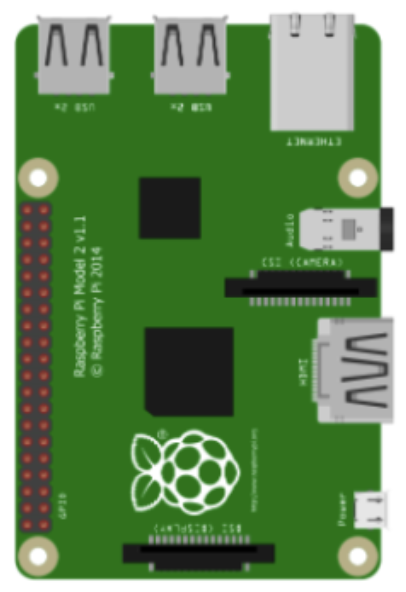
Overview
The Raspberry Pi is the control computer on the car. Using a python script, the raspberry pi will perform the PID control and obstacle avoidance algorithms and send instructions to the Arduino Mega. The raspberry pi also acts as the bridge for a user controlled Android app and the car.
It was decided to use a wifi connection to connect the Android and the Raspberry Pi. This provides a secure connection that is faster than bluetooth and be configured to our needs. Using the school’s wifi network is an option, however, setting up the Raspberry Pi as the access point is the ideal approach. Using the Raspberry Pi as the wifi host allows us to connect directly to the Raspberry Pi and not use a 3rd party to establish the connection. To do this the HOSTAPD and DNSMASQ libraries were installed.
Communicating with Android Phone
To communicate with the Android device the socket library was needed. This allows us to create a socket and read or write to it. Once the data is read from the socket, the python script decodes it from UTF-8 to interpret the message. This was done by sending a string of 2 numbers, the first one identified left right or both and the second identified if were turning it on. So 11 meant turn left, 21 meant turn right, 31 meant move forward and 30 meant stop. Once these interpretations are read in from the socket a conditional is then used to determine which function to use.
Bridging the Arduino and Android
The Android device writes to the socket and then the python script reads this, decodes and interprets the command as turn left, turn right start or stop. It then calls the respective function which translates to telling the Arduino what LEDs to turn on, flash or turn off. In this loop, if the Android device ever disconnect from the Raspberry Pi, the script goes to a stop the car state and then the script stops execution.
Run Time Changes
The first step was making it always run since the script stopped after the Android device disconnected. So when a phone disconnects, instead of stopping the script, it goes back into a listening state where it waits for a phone connection. The last step was making the script run as soon as the Raspberry Pi was turned on. In order to meet the constraints, the terminal was created and stored it in an autoStart folder in the Raspberry Pi’s .config folder. The last thing added was a log file that time stamped each operation performed so after several runs the team can debug any issues that occured.