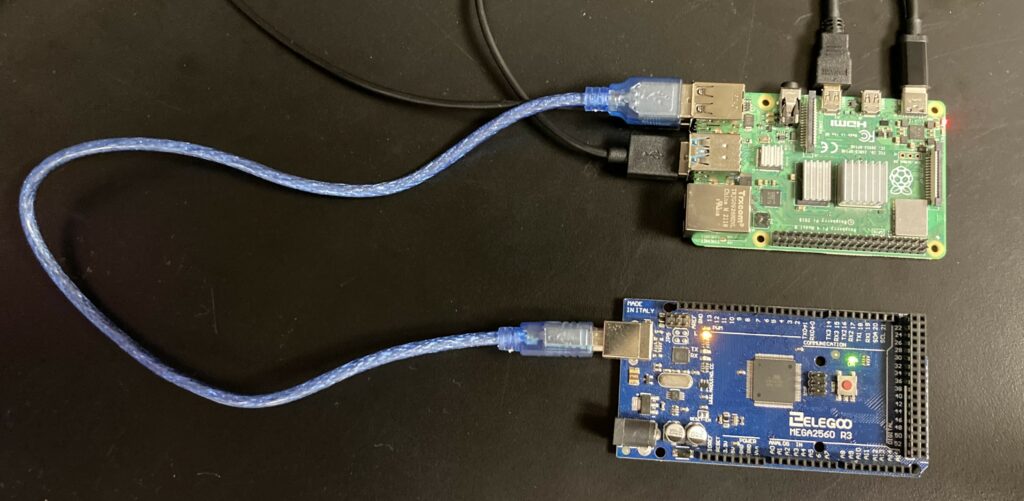
The group was able to send messages between the Raspberry Pi 4 and Arduino Mega via serial communication. A python script running on the Pi accepts a number from the command line and sends it to the Mega using a popular Python library for sending serial information called PySerial. The Mega then increments the number by 1 and sends it back to the Raspberry Pi which will display the new number to the terminal.
The serial.Serial(…) command on line 3 opens serial communication on port /dev/ttyACM0 because the Mega was connected to port /dev/ttyACM0 on the Pi. Notice how the baud rate on the Pi must match the baud rate on the Mega (115,200 Hz).
The terminal shows the result of running the Python code after uploading the Arduino .ino file to the Mega.
Python Code
import serial
import time
arduino = serial.Serial(port='/dev/ttyACM0', baudrate=115200, timeout=.1)
def write_read(x):
arduino.write(bytes(x, 'utf-8'))
time.sleep(0.05)
data = arduino.readline()
return data
while True:
num = input("Enter a number: ") # Taking input from user
value = write_read(num)
print(value) # printing the value
Arduino Code
int x;
void setup() {
Serial.begin(115200);
Serial.setTimeout(1);
}
void loop() {
while (!Serial.available());
x = Serial.readString().toInt();
Serial.print(x + 1);
}
Running PySerial in Raspberry Pi Terminal
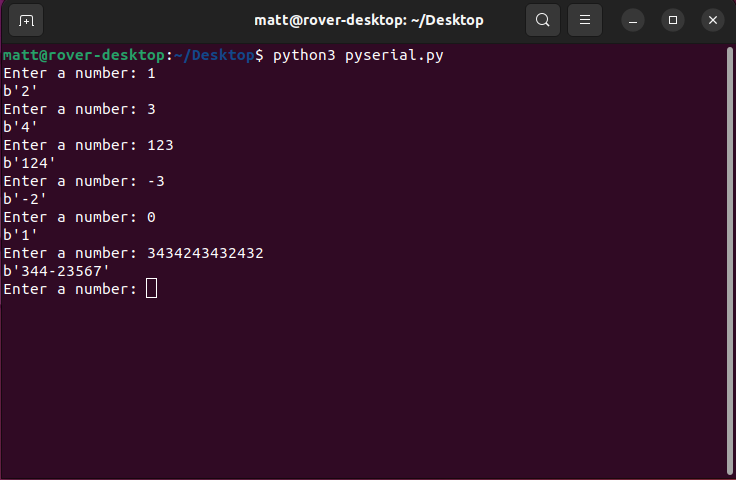